728x90
기존 Node.js에서는 HTTP 모듈로 서버 생성 → 요청 URL과 메서드에 따라 조건문으로 처리해야 했지만,
Express를 사용하면 app.get(), app.post() 등으로 간단하게 라우팅을 처리할 수 있다.
✅ 기존 Node.js 방식 (express 없이)
const http = require('http');
const server = http.createServer((req, res) => {
if (req.method === "GET" && req.url === "/") {
res.writeHead(200, { "Content-Type": "text/plain" });
res.end("Hello from Node.js!");
} else {
res.writeHead(404, { "Content-Type": "text/plain" });
res.end("Page not found.");
}
});
server.listen(3000, () => {
console.log("Server is running on port 3000");
});
✅ Express 사용 시
const express = require("express");
const app = express();
app.get("/", (req, res) => {
res.send("Hello from Express!");
});
app.listen(3000, () => {
console.log("Express server is running on port 3000");
});
app.use('/user/:id',(req,res,next)=>{
console.log('Request URL:',req.originalUrl);
next();
},(req,res,next)=>{
console.log('Request Type:', req.method);
next();
});
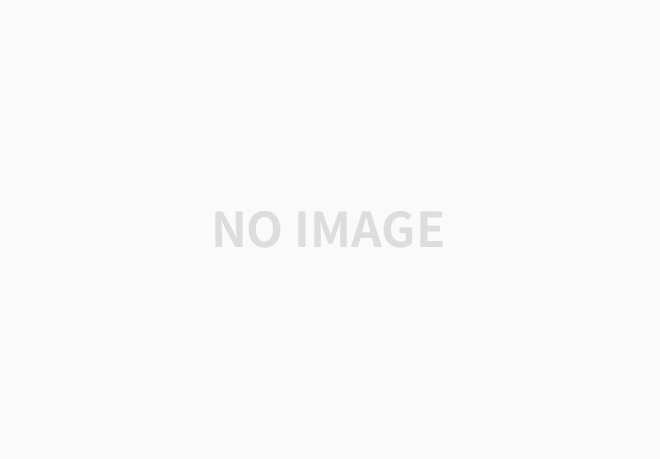
const port = 80;
const express = require("express");
const app = express();
app.get("/user/:id", (req, res, next) => {
if (req.params.id === '0') next('route'); // 다음 라우트로 이동
else next(); // 아래 미들웨어로 계속
}, (req, res, next) => {
res.send('regular'); // 일반 처리
});
app.get("/user/:id", (req, res, next) => {
res.send('special'); // id === '0'일 때만 실행됨
});
✅req.params란?
- req.params는 라우트 경로에 포함된 변수값들을 담고 있는 객체야.
- URL 경로에서 :변수이름으로 정의한 부분이 여기에 들어감.
app.get("/user/:id", (req, res) => {
console.log(req.params); // 👉 { id: '123' }
console.log(req.params.id); // 👉 '123'
res.send(`User ID is ${req.params.id}`);
});
🔗 클라이언트 요청:
GET /user/123
🔍 동작
- :id에 해당하는 '123'이 req.params.id로 들어
✅ 다중 파라미터 예제
app.get("/user/:userId/book/:bookId", (req, res) => {
console.log(req.params); // 👉 { userId: '7', bookId: '99' }
});
요청 URL
GET /user/7/book/99
→ req.params.userId === '7', req.params.bookId === '99'
언제 사용?
- /product/:id, /profile/:username처럼 URL 자체가 데이터를 담고 있을 때
- 폼을 통해 URL 이동하는 경우
- RESTful API 라우팅
728x90
'⚙️ Back-end > Node.js' 카테고리의 다른 글
[Node.js] passport.js 적용법 (0) | 2025.05.06 |
---|---|
[Node.js] 여러가지 초기 셋팅 및 DB 설정 (0) | 2024.11.21 |